<html>
<head>
<meta content="text/html; charset=ISO-8859-1" http-equiv="content-type">
<script type="application/javascript">
function bookStore()
{
var products={"Book":[{
"title": "Undestanding Jason",
"Author": "Isabelle",
"Price": 50,
"Discount": 5
},
{
"title": "Undestanding C++",
"Author": "Bells",
"Price": 150,
"Discount": 15
}
],
"DVD":[{
"title": "Undestanding HTML5",
"Price": 60,
"Discount": 7
},
{
"title": "Undestanding Javascript",
"Price": 100,
"Discount": 5
}
]
};
var tmp='';
for (var i=0; i<products.Book.length; i++)
tmp+=products.Book[i].title+'<br>'+products.Book[i].Author+'<hr>';
document.getElementById('list').innerHTML=tmp;
}
</script>
<title>JSON - Accessing from Javascript</title>
</head>
<body onload="bookStore();">
<div id="list"></div>
</body>
</html>
In the above example, the code in red is a JSON object that is assigned directly to the javascript variable products. Let's examine the JSON object in more detail:
{"Book":[{
"Title": "Undestanding JSON",
"Author": "Isabelle",
"Price": 50,
"Discount": 5
},
{
"Title": "Undestanding C++",
"Author": "Solomon",
"Price": 150,
"Discount": 15
}
],
"DVD":[{
"Title": "Undestanding HTML5",
"Price": 60,
"Discount": 7
},
{
"Title": "Undestanding Javascript",
"Price": 100,
"Discount": 5
}
]
}
In the above data structure, Book and DVD are two single-dimensional arrays. Book contains two array values. Each array value is an object with 4 members: Title, Author, Price and Discount. The array DVD also contains two values. Each array value is an object with 3 members: Title, Price and Discount. The data structure is assigned to the variable 'products'. Graphically, the datastructure looks as follows:
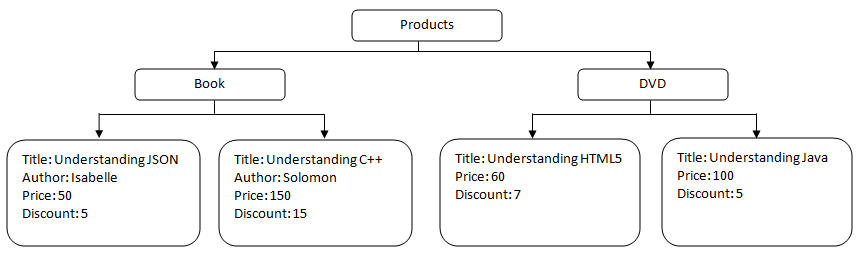
You can now access any member from the data structure just as you would a javascript object. For example. if you want to know the title of the first book, you can use products.Book[0].Title. To access the discount on the 'understanding HTML5' DVD, you can use products.DVD[0].Discount. The number of books can be accessed using products.Book.length
In our example, we are adding some HTML tags to the JSON data object members and displaying them inside a div tag.
var tmp='';
for (var i=0; i<products.Book.length; i++)
tmp+=products.Book[i].title+'<br>'+products.Book[i].Author+'<hr>';
document.getElementById('list').innerHTML=tmp;
</script>
<title>JSON - Accessing from Javascript</title>
</head>
<body onload="bookStore();">
<div id="list"></div>
</body>
</html>
To try out the example on this page, copy the code as is and paste it into a text editor like notepad. Save the file with the extension .htm. When you open the file from your browser, you should see the following output.
Output:
Understanding JSON
Isabelle
Understanding C++
Solomon
|